Color gradients and shades
#include "visual/Whiteboard.h"
#include "base/CommandLineParser.h"
#include "base/FileParser.h"
#include "base/SVector.h"
#include "visual/Color.h"
#include "visual/Axes.h"
#include <iostream>
int main( int argc, char** argv )
{
// Command line processing
commandArg<string> aStringO("-o","outfile (post-script)");
commandLineParser P(argc,argv);
P.SetDescription("Color scale example");
P.registerArg(aStringO);
P.parse();
string o = P.GetStringValueFor(aStringO);
// Define offset
double x_offset = 20;
double y_offset = 20;
int i, j;
// Declare whiteboard object
ns_whiteboard::whiteboard board;
double x_max = 0.;
double y_max = 0.;
double x = 0.;
double y = 0.;
int n = 100;
double w = 10.;
// Let's color this in on a scale from -1 to +1
for (i=-n; i<=n; i++) {
double v = (double)i/(double)n;
// Blue to red
color one = color(0.78, 0.1, 0.1);
color two = color(0.1, 0.1, 0.78);
// Get color gradient wuth white=0
color col = GradientMult(v, one, two);
x = n + i;
// Fill in the scale with a rectangle
board.Add( new ns_whiteboard::rect( ns_whiteboard::xy_coords(x + x_offset, y + y_offset + w),
ns_whiteboard::xy_coords((x+1) + x_offset, y + y_offset),
col) );
// Dumb way of keeping track how much space we need (x)
if (x > x_max)
x_max = x;
}
// Dumb way of keeping track how much space we need (y)
if (y + w > y_max)
y_max = y + w;
// Add spacer
y += 1.2*w;
// Add text to explain what we are looking at
board.Add( new ns_whiteboard::text( ns_whiteboard::xy_coords(x_offset, y + y_offset),
"Colour scale -1 to +1 (0 = white)", black, 6., "Times-Roman", 0, true));
// More spacer
y += w;
// Next color scale, green to red with black=0 (heat map style)
for (i=-n; i<=n; i++) {
double v = (double)i/(double)n;
color one = color(0., 0.99, 0.2);
color two = color(0.99, 0.1, 0.1);
color col = GradientMult(v, one, two, color(0, 0, 0));
x = n + i;
board.Add( new ns_whiteboard::rect( ns_whiteboard::xy_coords(x + x_offset, y + y_offset + w),
ns_whiteboard::xy_coords((x+1) + x_offset, y + y_offset),
col) );
if (x > x_max)
x_max = x;
}
if (y + w > y_max)
y_max = y + w;
y += 1.2*w;
board.Add( new ns_whiteboard::text( ns_whiteboard::xy_coords(x_offset, y + y_offset),
"Colour scale -1 to +1 (black)", black, 6., "Times-Roman", 0, true));
y += w;
n *=2;
// Simple gradient with two colors (dark red to light yellow)
for (i=0; i<=n; i++) {
double v = (double)i/(double)n;
color one = color(0.5, 0., 0.1);
color two = color(0.99, 0.99, 0.);
color col = Gradient(v, two, one);
x = i;
board.Add( new ns_whiteboard::rect( ns_whiteboard::xy_coords(x + x_offset, y + y_offset + w),
ns_whiteboard::xy_coords((x+1) + x_offset, y + y_offset),
col) );
if (x > x_max)
x_max = x;
}
if (y + w > y_max)
y_max = y + w;
y += 1.2*w;
board.Add( new ns_whiteboard::text( ns_whiteboard::xy_coords(x_offset, y + y_offset),
"Colour gradient", black, 6., "Times-Roman", 0, true));
// Get ready to write out the graph
ofstream out(o.c_str());
// Display it, and define the drawing area
ns_whiteboard::ps_display display(out, x_max + 2 * x_offset, y_max + 2 * y_offset);
// Write to file
board.DisplayOn(&display);
return 0;
}
Result:
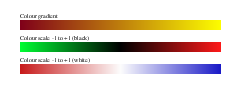