Simple compound objects
#include "visual/Whiteboard.h"
#include "base/CommandLineParser.h"
#include "base/FileParser.h"
#include "base/SVector.h"
#include "visual/Color.h"
#include "visual/Compounds.h"
#include <iostream>
int main( int argc, char** argv )
{
// Command line parsing
commandArg<string> aStringO("-o","outfile (post-script)");
commandLineParser P(argc,argv);
P.SetDescription("Using simple compound objects.");
P.registerArg(aStringO);
P.parse();
// Now we have the output name
string o = P.GetStringValueFor(aStringO);
// Offset at 20 dots
double x_offset = 20;
double y_offset = 20;
int i, j;
// Define the main object
ns_whiteboard::whiteboard board;
// Lets allocate some are to draw (in dots)
double x_max = 200.;
double y_max = 200.;
// Use the box class with default width (1.0) and color (black).
Box b;
// Let's draw a black rectangle!
b.Draw(board,
ns_whiteboard::xy_coords(20, 20),
ns_whiteboard::xy_coords(60, 60));
// Something a little bit more creative
b.Draw(board,
ns_whiteboard::xy_coords(30, 70),
ns_whiteboard::xy_coords(20, 120),
ns_whiteboard::xy_coords(60, 140),
ns_whiteboard::xy_coords(70, 75));
// Now arrows
Arrow a;
// From and to coordinates
a.Draw(board,
ns_whiteboard::xy_coords(110, 25),
ns_whiteboard::xy_coords(170, 25));
// One more
a.Draw(board,
ns_whiteboard::xy_coords(100, 30),
ns_whiteboard::xy_coords(150, 80));
// Let's change the angle of the arrow head
Arrow af(10., 0.);
af.Draw(board,
ns_whiteboard::xy_coords(100, 20),
ns_whiteboard::xy_coords(100, 110));
// Define output file
ofstream out(o.c_str());
// Define a display
ns_whiteboard::ps_display display(out, x_max + 2 * x_offset, y_max + 2 * y_offset);
// Save it to a postscript file.
board.DisplayOn(&display);
return 0;
}
Result:
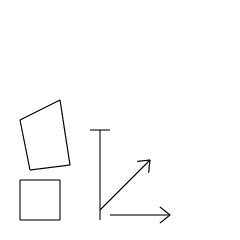