Simple 3D geometry
#include "visual/Whiteboard.h"
#include "base/CommandLineParser.h"
#include "base/FileParser.h"
#include "base/SVector.h"
#include "visual/Color.h"
#include "visual/Axes.h"
#include "visual/Geometry.h"
#include <iostream>
// Draw three lines in a 3d geometry
void Draw(const string & o, double x, double y)
{
// Offset
double x_offset = 20;
double y_offset = 20;
int i, j;
// Declare the whiteboard
ns_whiteboard::whiteboard board;
// Set drawing area
double x_max = 300.;
double y_max = 300.;
// Add background (only needed for later conversion to certain other graphics formats)
board.Add( new ns_whiteboard::rect( ns_whiteboard::xy_coords(0, 0),
ns_whiteboard::xy_coords(x_max + 2*x_offset, y_max + 2*y_offset),
color(0.99,0.99,0.99)));
// Declare geometry
Geometry3D g;
// Move the center to 150
g.SetOffset(150.);
// Set the angle from which we look at it
g.SetRotation(x, y);
// Length of the lines
double d = 170.;
y = 0.;
// Add three lines in different colors
board.Add( new ns_whiteboard::line( g.Coords(0, 0, 0),
g.Coords(d, 0, 0),
1.,
color(0.99,0.,0.)));
board.Add( new ns_whiteboard::line( g.Coords(0, 0, 0),
g.Coords(0, d, 0),
1.,
color(0.,0.99,0.)));
board.Add( new ns_whiteboard::line( g.Coords(0, 0, 0),
g.Coords(0, 0, d),
1.,
color(0.,0.,0.99)));
// Open output stream
ofstream out(o.c_str());
// Declare a post-script display
ns_whiteboard::ps_display display(out, x_max + 2 * x_offset, y_max + 2 * y_offset);
// Display & save...
board.DisplayOn(&display);
}
// Main routine
int main( int argc, char** argv )
{
commandArg<string> aStringO("-o","outfile (post-script)");
commandLineParser P(argc,argv);
P.SetDescription("Simple 3d plot");
P.registerArg(aStringO);
P.parse();
string o = P.GetStringValueFor(aStringO);
Draw(o, 0.3, 0.0);
return 0;
}
Result:
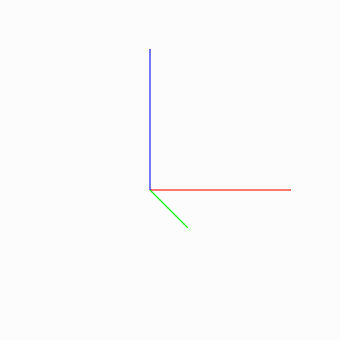